Your company has settled on Plivo to handle its voice and messaging communications, and now it’s your job to start integrating Plivo into your company’s applications. Don’t worry — Plivo has a Node.js SDK to help you out. You can use it write Node.js applications that send and receive SMS messages.
In this guide, we’ll outline the steps for getting set up to use Node.js and Express.js in Plivo’s SMS API. Start by setting up your Node.js environment and connecting your Plivo account. We’ve also got code snippets for you to leverage to send and receive messaging through our SMS API. By the end, you'll be ready to leverage SMS communication for notifications, alerts, or even two-way interactions in Node.js.
Prerequisites
To send SMS with Node.js, you need the following elements in place:
- A Plivo account - If you don't have one already, sign up with your work email address.
- Install Node
- Install Plivo’s Node SDK
- Install the Express framework.
If this is your first time using Plivo APIs, follow our instructions to set up a Node.js development environment. Once you’re completed these steps, carry on to learn how to send SMS in Node.js.
Send SMS in Node.js
Create a file called SendSMS.js and paste this code into it.
Replace the auth placeholders with actual values from the Plivo console. Replace the phone number placeholders with actual phone numbers in E.164 format (for example, +12025551234). In countries other than the US and Canada you can use a sender ID for the message source. You must have a Plivo phone number to send messages to the US or Canada; you can rent a Plivo number from Phone Numbers >Buy Numbers on the Plivo console or via the Numbers API. Save the file and run it.
Note: If you’re using a Plivo Trial account, you can send messages only to phone numbers that have been verified with Plivo. You can verify (sandbox) a number by going to the console’s Phone Numbers > Sandbox Numbers page.
Receive SMS in Node.js
Of course, sending messages is only half of the equation. Plivo supports receiving SMS text messages in many countries (see our SMS API coverage page and click on the countries you’re interested in). When someone sends an SMS message to a Plivo phone number, you can receive it on your server by setting a Message URL in your Plivo application. Plivo will send the message along with other parameters to your Message URL.
Create a file called receive_sms.js (or whatever name you like) and paste into it this code.
Save the file and run it.
You should then be able to see your basic server app in action on http://localhost:3000/receive_sms/.
That’s fine for testing, but it’s not much good if you can’t connect to the internet to receive incoming messages and handle callbacks. For that, we recommend using ngrok, which exposes local servers behind NATs and firewalls to the public internet over secure tunnels. Install it and run ngrok on the command line, specifying the port that hosts the application on which you want to receive messages.
Ngrok will display a forwarding link that you can use as a webhook to access your local server using the public network.
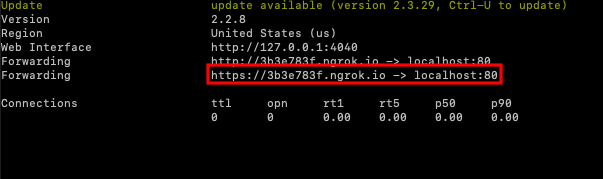
Now you can create an application to receive SMS messages (follow our Quickstart guide for details).
Why use Node.js to send and receive SMS
Node.js's event-driven, non-blocking architecture is well-suited for handling SMS communications. It excels at handling multiple concurrent SMS requests without blocking the application and can efficiently manage high volumes of SMS traffic.
Applications that use Node.js for backend benefit from all the pros of full-stack JavaScript development, including better efficiency, code sharing and reusability, speed, and productivity. Node.js is well-suited for applications requiring real-time SMS interactions, such as two-factor authentication or live chat.
Get started with Plivo to send SMS in Node.js
That’s all there is to sending and receiving SMS messages using Plivo’s Node.js SDK.
Don’t use Node.js? Don’t worry — we have SDKs for Java, Python, PHP, Ruby, .NET Core, .NET Framework, and Go.
Haven’t tried Plivo yet? Getting started is easy and only takes 5 minutes. Sign up today.