Once you’ve chosen Plivo to handle your company’s voice and messaging channels, it’s time to start integrating Plivo’s tools into your company’s applications. We make this process easy with a .NET SDK to help you out. Use it to write .NET applications that send and receive SMS messages.
In this guide, we’ll explain how to send and receive SMS messages using .NET. We'll walk you through how to up your account, write code snippets for sending messages, and receive responses through our SMS API. By the end, you'll be ready to leverage SMS communication for notifications, alerts, or even two-way interactions in .NET.
Prerequisites
Before you dive into the steps to set up Plivo’s SMS API with ASP.NET Core, make sure the following prerequisites are in place.
- A Plivo account— sign up with your work email address if you don’t have one already.
- Install .NET Framework 4.6 or higher
- Install the Plivo .NET SDK using Visual Studio
- Trigger an API request
- Set up a .NET Framework application
- Set up ngrok
The prerequisites listed above are part of the instructions to set up a .NET development environment. If this is your first time using Plivo APIs, follow our guide to set up your dev environment to send and receive SMS messages.
Send an SMS message with ASP.NET Core
Now you’re ready to start. Open the file Program.cs in the CS project and paste into it this code.
Replace the auth placeholders with actual values from the Plivo console. Replace the phone number placeholders with actual phone numbers in E.164 format (for example, +12025551234). In countries other than the US and Canada you can use a sender ID for the message source. You must have a Plivo phone number to send messages to the US or Canada; you can rent a Plivo number from Phone Numbers >Buy Numbers on the Plivo console or via the Numbers API. Save the file and run it.
Note: If you’re using a Plivo Trial account, you can send messages only to phone numbers that have been verified with Plivo. You can verify (sandbox) a number by going to the console’s Phone Numbers > Sandbox Numbers page.
Receive an SMS message with ASP.NET Core MVC
Of course sending messages is only half of the equation. Plivo supports receiving SMS text messages in many countries (see our SMS API coverage page and click on the countries you’re interested in). When someone sends an SMS message to a Plivo phone number, you can receive it on your server by setting a Message URL in your Plivo application. Plivo will send the message along with other parameters to your Message URL.
Begin by setting up a .NET Core app. Create a new project directory.
Change to that directory and initialize the model-view-controller (MVC) architecture with the command
Change to the Controllers directory. Create a controller named ReceiveSmsController.cs and paste into it this code.
Before you can test the application, edit Properties/launchSettings.json and set the applicationUrl.
Then start the local server with the command
You should then be able to see your basic server app in action on http://localhost:5000/receivesms/.
That’s fine for testing, but it’s not much good if you can’t connect to the internet to receive incoming messages and handle callbacks. For that, we recommend using ngrok, which exposes local servers behind NATs and firewalls to the public internet over secure tunnels. Install it and run ngrok on the command line, specifying the port that hosts the application on which you want to receive messages.
Ngrok will display a forwarding link that you can use as a webhook to access your local server using the public network.
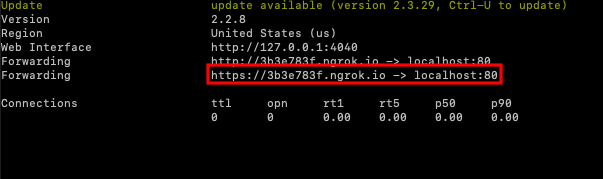
Now you can create an application to receive SMS messages (follow our Quickstart guide for details).
Why integrate Plivo’s SMS API with ASP.NET?
ASP.NET provides a strong foundation for integrating Plivo’s SMS API with your business tech stack. Many developers are already well-versed in C# and Visual Studio, making it faster and easier to leverage this framework for your web application.
Keeping your SMS logic within your ASP.NET application simplifies code management and maintenance. Everything is in one place. And, it scales well for handling high volumes of SMS traffic. Beyond SMS, ASP.NET allows you to integrate with other functionalities like email, databases, and user authentication, creating a powerful web application ecosystem.
Conclusion
That’s all there is to sending and receiving SMS messages using Plivo’s .NET Core SDK. Don’t use .NET Core? Don’t worry — we have SDKs for Java, Python, PHP, Node.js, Ruby, and Go.
Haven’t signed up for Plivo yet? Getting started is easy and only takes five minutes. Sign up today.