Your company has chosen Plivo to handle its voice and messaging communications. Now, it’s your job to integrate Plivo into your company’s applications. Don’t worry — you can send SMS in PHP using the PHP SDK and easily write PHP applications that send and receive SMS messages.
This guide will explain the steps for sending and receiving SMS messages in PHP. We'll cover everything from setting up your account to crafting the code snippets for sending messages and handling incoming replies. By the end, you'll be ready to leverage SMS communication for notifications, alerts, or even two-way interactions within your PHP applications.
Prerequisites
Before diving into the steps in this guide, make sure you have the following prerequisites ready to go.
- A Plivo account — sign up with your work email address if you don’t have one already.
- Install PHP
- Install Composer
- Install Laravel and create a Laravel project
- Install the PHP Plivo SDK
Send an SMS in PHP
Now you’re ready to start. Create a file called SendSMS.php and paste into it this code.
Replace the auth placeholders with actual values from the Plivo console. Replace the phone number placeholders with actual phone numbers in E.164 format (for example, +12025551234). In countries other than the US and Canada you can use a sender ID for the message source. You must have a Plivo phone number to send messages to the US or Canada; you can rent a Plivo number from Phone Numbers > Buy Numbers on the Plivo console or via the Numbers API. Save the file and run it.
Note: If you’re using a Plivo Trial account, you can send messages only to phone numbers that have been verified with Plivo. You can verify (sandbox) a phone number by going to the console’s Phone Numbers > Sandbox Numbers page.
Receive SMS in PHP
Of course sending messages is only half of the equation. Plivo supports receiving SMS text messages in many countries (see our SMS API coverage page and click on the countries you’re interested in). When someone sends an SMS message to a Plivo phone number, you can receive it on your server by setting a Message URL in your Plivo application. Plivo will send the message along with other parameters to your Message URL.
Create a file called receive_sms.php (or whatever name you like) and paste into it this code.
Save the file and run it.
You should then be able to see your basic server app in action at http://localhost:8000/receive_sms/.
That’s fine for testing, but it’s not much good if you can’t connect to the internet to receive incoming messages and handle callbacks. For that, we recommend using ngrok, which exposes local servers behind NATs and firewalls to the public internet over secure tunnels. Install it and run ngrok on the command line, specifying the port that hosts the application on which you want to receive messages.
Ngrok will display a forwarding link that you can use as a webhook to access your local server using the public network.
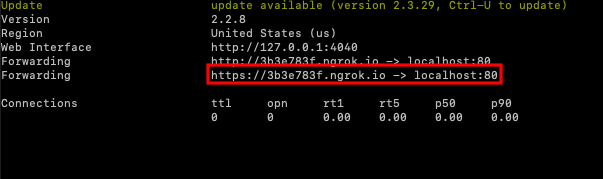
Now you can create an application to receive SMS messages (follow our Quickstart guide for details).
Best practices for sending SMS with PHP
Make sure your SMS messages are received and read by following these best practices.
1. Send using a valid phone number
Use a phone number that is registered with your gateway provider or a valid number associated with your account.
2. Use E.164 formatting
E.164 is an international standard numbering format developed for telephone systems worldwide. Numbers are a maximum of 15 digits starting with a plus sign (+) followed by the country code (e.g. 1 for the U.S.) and the mobile number.
3. Keep messages concise
Stick to around 160 characters to stay within GSM-7 encoding limits.
4. Provide an opt-out option
The Cellular Telecommunications Industry Association’s (CTIA) guidelines require brands to give their audience a way to opt-out of receiving future text messages (such as, “reply STOP to stop receiving these messages”).
To learn more about sending SMS with PHP, check out our developer documentation.
Get started with Plivo to send SMS in PHP
And that’s all there is to sending and receiving SMS messages using Plivo’s PHP SDK. Don’t use PHP? Don’t worry — we have SDKs for Java, Python, Node.js, Ruby, .NET Core, .NET Framework, and Go.
Haven’t tried Plivo yet? Getting started is easy and takes only five minutes. Sign up today.